Hello,
The thing is that by default, Adaxes creates home directories using credentials of Adaxes default service administrator. However, if the default service administrator does not have sufficient permissions to list the contents of the network share where the home directory needs to be created, Adaxes attempts to create a home directory using credentials of the user account used to logon to the domain. In your case, it looks like the default service administrator does not have sufficient permissions for the network share, and credentials of the domain logon account are used to create home directories.
To remedy the issue, you need to run the following PowerShell script after creating the home directory. It will remove permissions for the domain logon account.
Import-Module Adaxes
# Get home directory path
try
{
$homeDirectoryPath = $Context.TargetObject.Get("homeDirectory")
}
catch
{
$Context.LogMessage("The user doesn't have a home directory", "Warning")
return
}
# Bind to the Configuration Set Settings container
$configSetSettingsPath = $Context.GetWellKnownContainerPath("ConfigurationSetSettings")
$configSetSettings = $Context.BindToObject($configSetSettingsPath)
# Get Security Identifier of the service administrators
$adminManager = $configSetSettings.AdministratorManager
$adminsSidsBytes = $adminManager.Administrators
$adminSids = New-Object "System.Collections.Generic.HashSet[System.String]"
foreach ($sidBytes in $adminsSidsBytes)
{
$sid = New-Object "Softerra.Adaxes.Adsi.Sid" @($sidBytes, 0)
[void]$adminSids.Add($sid)
}
# Get user SIDs of user account used for access to the domain
$domainName = $Context.GetObjectDomain("%distinguishedName%")
$managedDomainsPath = $Context.GetWellKnownContainerPath("ManagedDomains")
$managedDomainsPathObj = New-Object "Softerra.Adaxes.Adsi.AdsPath" $managedDomainsPath
$managedDomainPath = $managedDomainsPathObj.CreateChildPath("DC=$domainName")
$managedDomain = $Context.BindToObject($managedDomainPath)
$logonName = $managedDomain.LogonName
if (-not([System.String]::IsNullOrEmpty($logonName)))
{
$user = Get-AdmUser -Filter {userPrincipalName -eq $logonName} -AdaxesService localhost -Server $domainName -ErrorAction SilentlyContinue
if ($user -ne $NULL)
{
[void]$adminSids.Add($user.Sid)
}
}
# Get home directory Access Control List
$acl = Get-Acl -Path $homeDirectoryPath
# Find and remove administrative users from the Access Control List
$accessRules = $acl.Access
for ($i = $accessRules.Count - 1; $i -ge 0; $i--)
{
$accessRule = $accessRules[$i]
$isInherited = $accessRule.IsInherited
foreach ($identityReference in $accessRule.IdentityReference)
{
# Translate identity to SID
$sid = $identityReference.Translate("System.Security.Principal.SecurityIdentifier").Value
if (!($adminSids.Contains($sid)))
{
continue
}
# Check whether permissions are inherited from a parent container
$userIdentity = $identityReference.Value
$userPermissions = $identityReference
if ($isInherited)
{
$Context.LogMessage("Cannot remove permissions '$userPermissions' for '$userIdentity' because the access rule is inherited from a parent container", "Warning")
continue
}
[void]$acl.RemoveAccessRule($accessRule)
}
}
# Assign the modified Access Control List
$folder = Get-Item $homeDirectoryPath
$folder.SetAccessControl($acl)
For this purpose, add the Run a program or PowerShell script action to the Business Rule that creates the home directories immediately after the action that creates home directories.
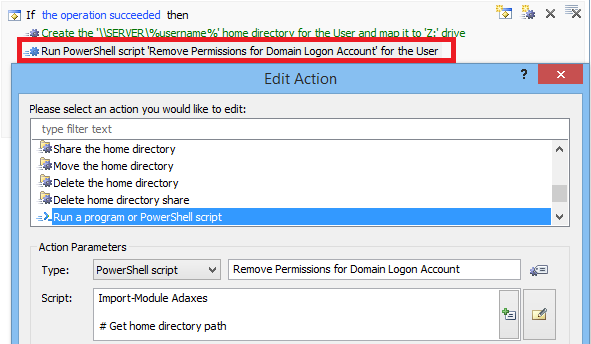