Hello,
Yes, there is. You can create a Business Rule executed before creating a user that will cancel the operation if a password does not meet the complexity requirements. To check for complexity requirements, you'll need to run a PowerShell script.
To create such a Business Rule:
-
Create a new Business Rule.
-
On the 2nd step of the Create Business Rule wizard, select User and Before Creating a User.
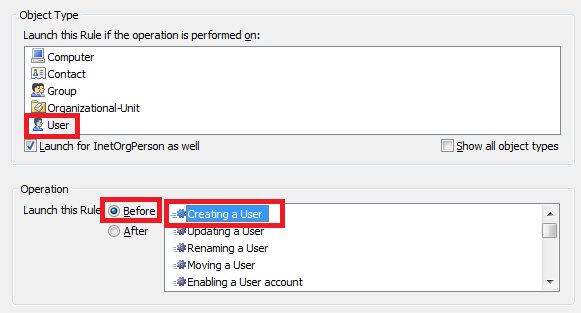
-
On the 3rd step, add the Run a program or PowerShell script action and paste a script that will do the job.
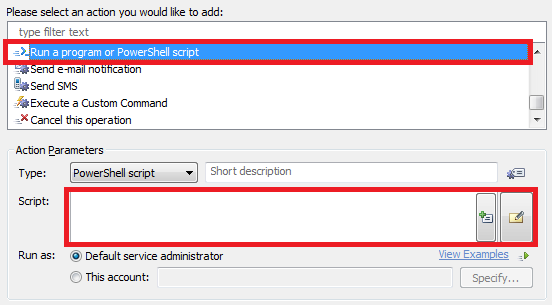
For example, here's a script that checks whether a new user's password meets the Password Complexity Requirements defined by Microsoft. Also, it checks whether the password length is at least 7 characters (defined by $minLength).
$minLength = 7 # TODO: modify me
$minRequirementsToPass = 3 # TODO: modify me
$requirements = @{
[regex]"[A-Z]" = 1, "The password does not contain an upper-case character.";
[regex]"[a-z]" = 1, "The password does not contain a lower-case character.";
[regex]"[0-9]" = 1, "The password does not contain a number.";
[regex]"[^a-zA-Z0-9]" = 1, "The password does not contain a special character."}
# TODO: modify me. Example: @{<requirement> = <minimum number of characters>, "Error message"}
if ($Context.IsPasswordChanged())
{
$password = $Context.GetNewPassword();
# Check password length
if($password.length -lt $minLength)
{
$Context.Cancel("The password does not have at least $minLength characters.")
return
}
# Passwords must not contain the user's entire samAccountName (Account Name) value.
$username = "%username%".ToLower()
if ($password.ToLower().Contains($username.SubString(0,3)))
{
$Context.Cancel("The password should not contain the username or parts of it.")
return
}
# Passwords must not contain the user's entire displayName (Full Name) value
$displayName = $Context.GetModifiedPropertyValue("displayName")
$delimiters = @(".", ",", "-", "_", " ", "#")
$displayNameParts = $displayName.Split($delimiters)
foreach ($string in $displayNameParts)
{
if ($string.length -lt 3)
{
continue
}
if ($password.ToLower().Contains($string.ToLower()))
{
$Context.Cancel("The password should not contain the user's Display Name or parts of it.")
return
}
}
# Check whether the password meets at least three complexity requirements
$requirementsPassed = 0
$errorMessages = @()
foreach ($requirement in $requirements.Keys)
{
$minNubmerCharacters = ($requirements[$requirement])[0]
if ($requirement.Matches($password).Count -lt $minNubmerCharacters)
{
$errorMessages += ($requirements[$requirement])[1]
continue
}
$requirementsPassed++
}
# If the password does not meet at least three requirements, cancel operation.
if ($requirementsPassed -lt $minRequirementsToPass)
{
$requirementsLeft = $minRequirementsToPass - $requirementsPassed
$requirementsCount = $requirements.Count
$Context.Cancel("The password must meet at least $minRequirementsToPass out of $requirementsCount complexity requirements of which only $requirementsPassed has been met. Meet at least $requirementsLeft more of the requirements above.")
foreach ($message in $errorMessages)
{
$Context.LogMessage($message, "Error")
}
return
}
}