Hello,
Essentially, we'd like to see if a computer name contains a username, and if the department of that user = IT for instance, move the computer object into the comp_IT group.
This can be easily achieved with the help of a PowerShell script. For example the following script can be used to locate a computer object whose name contains the username of the user on which the script is executed. If such a computer is found, the script adds it to the group that corresponds to the user's department.
Also, you can create a Scheduled Task that will run the script on a certain periodic basis to keep in sync with changes in your AD. For information on how to create a Scheduled Task, see the following tutorial: http://www.adaxes.com/tutorials_Automat ... gement.htm. To add a script to a Scheduled Task, use the Run a program or PowerShell script action.
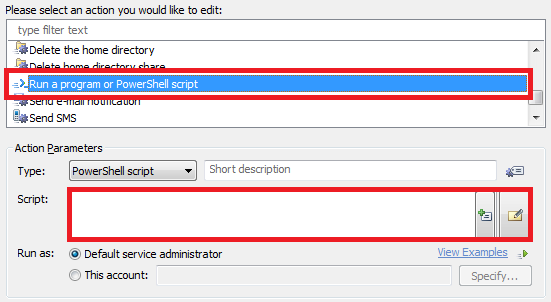
In the script, $departmentInfos specifies a hash table of all the departments and matching AD groups.
The script:
$departmentInfos = @{
"Sales" = "Comp_Slaes"
"IT" = "Comp_IT"
} # TODO: modify me. Example $departmentInfos = @{"<department name>" = "<group_name>"}
function GetObjectPath($filter, $domainName)
{
$searcher = $Context.BindToObject("Adaxes://$domainName/rootDSE")
$searcher.SearchFilter = $filter
$searcher.SearchScope = "ADS_SCOPE_SUBTREE"
$searcher.PageSize = 500
try
{
$searchResult = $searcher.ExecuteSearch()
$objects = $searchResult.FetchAll()
if ($objects.Count -eq 0)
{
return $NULL
}
return $objects[0].AdsPath
}
finally
{
$searchResult.Dispose()
}
}
# Get the user's computer path
$domainName = $Context.GetObjectDomain("%distinguishedName%")
$computerPath = GetObjectPath "(&(objectCategory=computer)(sAMAccountName=*%username%*))" $domainName
if ($computerPath -eq $NULL)
{
$Context.LogMessage("A user's computer could not be found", "Warning")
return
}
# Search group matching the department
$groupName = $departmentInfos["%department%"]
if ($groupName -eq $NULL)
{
$Context.LogMessage("No group specifieded for department '%department%'", "Warning")
return
}
$groupPath = GetObjectPath "(&(objectCategory=group)(sAMAccountName=$groupName))" $domainName
if ($groupPath -eq $NULL)
{
$Context.LogMessage("Group '$groupName' does not exist", "Warning")
return
}
# Add the computer to group
$group = $Context.BindToObject($groupPath)
$group.Add($computerPath)