Hello,
There is no built-in action to delete a user's profile in Adaxes, but you can always use a script for this purpose. Here's a script that deletes a user's standard profile, V2 profile and V4 profile (appears in Windows 8 / Server 2012):
$profileSuffixes = @(".V2", ".V4") # TODO: modify me
# Get name of the user who invokes the script
$adminName = "$env:userdomain\$env:username"
# Function to get full access to all subdirectories in a directory
function GrantFullControlForDirectory($directoryPath, $username, $directoryWithFullPermission)
{
if ($directoryWithFullPermission.Contains($directoryPath))
{
return
}
$directoryWithFullPermission.Add($directoryPath) | Out-Null
$directory = Get-Item -Path $directoryPath -Force
# Change directory owner
$ownerAcl = New-Object "System.Security.AccessControl.DirectorySecurity"
$ownerID = New-Object "System.Security.Principal.NTAccount" $username
$ownerAcl.SetOwner($ownerID)
$directory.SetAccessControl($ownerAcl)
# Set the Full Access permission
$directoryAcl = Get-Acl $directoryPath
$fullPermission = New-Object "System.Security.AccessControl.FileSystemAccessRule" $userName, "FullControl","ContainerInherit, ObjectInherit", "None", "Allow"
$directoryAcl.SetAccessRule($fullPermission)
Set-Acl -Path $directoryPath -AclObject $directoryAcl
$childItems = Get-ChildItem -Path $directoryPath -Force
if($childItems -eq $NULL)
{
return
}
foreach($item in $childItems)
{
if($item -is [System.IO.DirectoryInfo])
{
GrantFullControlForDirectory $item.FullName $username $directoryWithFullPermission
}
}
}
# Function to get full access to all files in a directory
function GrantFullControlForFiles($directoryPath, $username)
{
# Get full access to all files in the directory
$allFilePaths = [System.IO.Directory]::GetFiles($directoryPath,"*","AllDirectories")
foreach($filePath in $allFilePaths)
{
$file = Get-Item -Path $filePath -Force
# Change owner
$ownerAcl = New-Object "System.Security.AccessControl.FileSecurity"
$ownerID = New-Object "System.Security.Principal.NTAccount" $username
$ownerAcl.SetOwner($ownerID)
$file.SetAccessControl($ownerAcl)
# Set Full Access permission
$fileAcl = Get-Acl $filePath
$fullPermission = New-Object "System.Security.AccessControl.FileSystemAccessRule" $username, "FullControl", "Allow"
$fileAcl.SetAccessRule($fullPermission)
Set-Acl -Path $filePath -AclObject $fileAcl
}
}
# Get profile path from AD
$profilePaths = @()
try
{
$profilePath += $Context.TargetObject.Get("profilePath")
$profilePaths += $profilePath
}
catch
{
$Context.LogMessage("No profile path specified", "Information") # TODO: modify me
return
}
# Also check profile paths with suffixes (V2 and V4 profiles)
foreach ($suffix in $profileSuffixes)
{
$profilePaths += "$profilePath$suffix"
}
# Remove profile folders
foreach ($profilePath in $profilePaths)
{
if (!(Test-Path -Path $profilePath))
{
$Context.LogMessage("Profile '$profilePath' missing", "Information")
continue
}
# Change permissions
$directoryWithFullPermission = New-Object "System.Collections.Generic.HashSet[System.String]"
GrantFullControlForDirectory $profilePath $adminName $directoryWithFullPermission
GrantFullControlForFiles $profilePath $adminName
# Remove the profile folder
try
{
Remove-Item -Path $profilePath -Force -Recurse -ErrorAction Stop
$Context.LogMessage("Profile '$profilePath' successfully removed", "Information")
}
catch
{
$Context.LogMessage($_.Exception.Message, "Error") # TODO: modify me
}
}
To add the script to your Business Rule, Custom Command or Scheduled Task, use the Run a program or PowerShell script action.
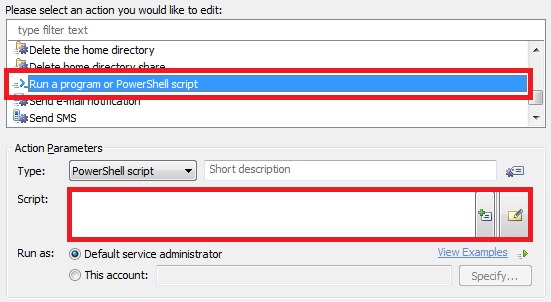